Python-ticks
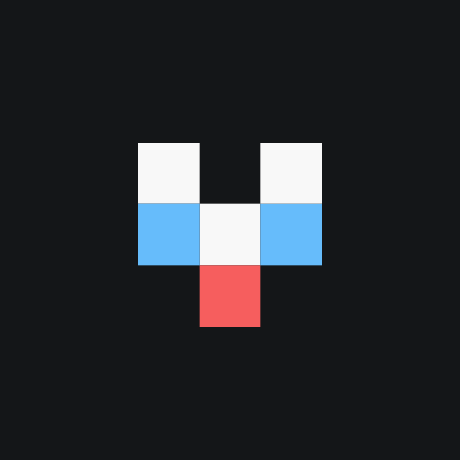
整理和维护经常会用到的python技巧
- Upack
当需要从某个可迭代对象中分决出N个元素,但是这个可迭代对象的长度可能超过N,这会导致“分解的值过多(too many values to unpack)”的异常
使用python的“*表达式”可以解决这个问题。
1 |
|
在分解任意长度的可迭代对象时特别好用
1 |
|
- 步进切片
从列表中创建一定间隔的新列表
1 |
|
list slice with step (a[start:end:step])
- 生成倒叙的list
1 |
|
命名切片的调用
Naming slices (slice(start,end,step))1
2
3
4
5
6>>> a = [0, 1, 2, 3, 4, 5]
>>> LASTTHREE = slice(-3, None)
>>> LASTTHREE
slice(-3, None, None)
>>> a[LASTTHREE]
[3, 4, 5]遍历列表的索引和值
Iterating over list index and value pairs (enumerate)
1 |
|
- 遍历字典
dict.iteritems
1 |
|
压缩和解压缩列表
Zipping and unzipping lists1
2
3
4
5
6
7>>> a = [1, 2, 3]
>>> b = ['a', 'b', 'c']
>>> z = zip(a, b)
>>> z
[(1, 'a'), (2, 'b'), (3, 'c')]
>>> zip(*z)
[(1, 2, 3), ('a', 'b', 'c')]使用zip分组相邻的列表项
Grouping adjacent list items using zip
1 |
|
- 使用滑动窗口迭代器
Sliding windows (nn -grams) using zip and iterators
1 |
|
字典的键值反转
Inverting a dictionary using zip1
2
3
4
5
6
7
8>>> m = {'a': 1, 'b': 2, 'c': 3, 'd': 4}
>>> m.items()
[('a', 1), ('c', 3), ('b', 2), ('d', 4)]
>>> zip(m.values(), m.keys())
[(1, 'a'), (3, 'c'), (2, 'b'), (4, 'd')]
>>> mi = dict(zip(m.values(), m.keys()))
>>> mi
{1: 'a', 2: 'b', 3: 'c', 4: 'd'}展开列表
Flattening lists
1 |
|
- 生成器表达式
Generator expressions
1 |
|
- 字典推导式
Dicitonary comprehensions
1 |
|
- 使用字典推到式反转字典
Inverting a dictionary using a dictionary comprehension
1 |
|
- 命名元组的调用
Named tuples (collections.namedtuple)
1 |
|
- 从命名元组继承
inheriting from named tuples
1 |
|
- 集合的使用
sets and set operations
1 |
|
- 集合的操作
1 |
|
- 可迭代对象的计数
1 |
|
- 双端队列
double-ended queue
1 |
|
- 最大长度双端队列
double-ended queue with maximum length
1 |
|
- 有序字典
ordered dictionaries
1 |
|
将对象映射到唯一的计数数字
mapping objects to unique counting numbers1
2
3
4
5
6
7
8
9
10
11
12>>> import itertools, collections
>>> value_to_numeric_map = collections.defaultdict(itertools.count().next)
>>> value_to_numeric_map['a']
0
>>> value_to_numeric_map['b']
1
>>> value_to_numeric_map['c']
2
>>> value_to_numeric_map['a']
0
>>> value_to_numeric_map['b']
1最大和最小元素
largest and smallest elements(heapq.nlargest and heapq.nsmallest)1
2
3
4
5>>> a = [random.randint(0, 100) for __ in xrange(100)]
>>> heapq.nsmallest(5, a)
[3, 3, 5, 6, 8]
>>> heapq.nlargest(5, a)
[100, 100, 99, 98, 98]笛卡尔积
cartesian products1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26>>> for p in itertools.product([1, 2, 3], [4, 5]):
(1, 4)
(1, 5)
(2, 4)
(2, 5)
(3, 4)
(3, 5)
>>> for p in itertools.product([0, 1], repeat=4):
... print ''.join(str(x) for x in p)
...
0000
0001
0010
0011
0100
0101
0110
0111
1000
1001
1010
1011
1100
1101
1110
1111组合和替换组合
combinations and combinations with replacemnet
1 |
|
- 排列交换
Permurtations
1 |
|
- 链式迭代
chaining iterables1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33>>> a = [1, 2, 3, 4]
>>> for p in itertools.chain(itertools.combinations(a, 2), itertools.combinations(a, 3)):
... print p
...
(1, 2)
(1, 3)
(1, 4)
(2, 3)
(2, 4)
(3, 4)
(1, 2, 3)
(1, 2, 4)
(1, 3, 4)
(2, 3, 4)
>>> for subset in itertools.chain.from_iterable(itertools.combinations(a, n) for n in range(len(a) + 1))
... print subset
...
()
(1,)
(2,)
(3,)
(4,)
(1, 2)
(1, 3)
(1, 4)
(2, 3)
(2, 4)
(3, 4)
(1, 2, 3)
(1, 2, 4)
(1, 3, 4)
(2, 3, 4)
(1, 2, 3, 4)
- 按给定键进行分组
grouping rows by a given key
1 |
|
- 在任意目录中启动http服务器
start a static http server in any directory
1 |
|
- 本文标题:Python-ticks
- 本文作者:Roy
- 创建时间:2019-12-25 08:53:55
- 本文链接:https://www.yrzdm.com/2019/12/25/decomposing-elements-from-iteratable-objects-md/
- 版权声明:本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!