Python 推送信息到wechat
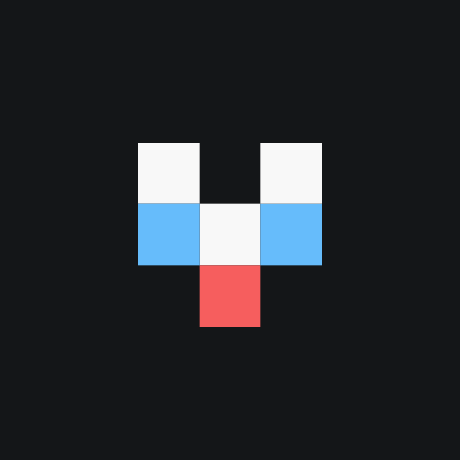
前言
最近看到网上有定时推送文章到微信的文章,其实这个小功能我一直在做,包括定时爬取内容用邮件发送等等。
不过网上都是python2.7的版本。对于使用python3的小白可能用起来比较困惑,手动改了一个python3
的版本。
#实现环境
- python3.6(conda)
- 数据源:爱词霸
- 调用地址:http://open.iciba.com/dsapi/
- 请求方式:GET
请求参数:
参数 | 必选 | 类型 | 说明 | ||
---|---|---|---|---|---|
date | 否 | string | 格式为:2018-08-01;默认取当天 | ||
type | 否 | string | 可选值为last和next;以date日期为准的last返回前一天,next返回后一天。 | ||
代码示例 | |||||
|
|||||
返回类型:JSON (JSON字段解释:) |
属性名 | 属性值类型 | 说明 |
---|---|---|
sid | string | 每日一句id |
tts | string | 音频地址 |
content | string | 英文内容 |
note | string | 中文内容 |
love | string | 每日一句喜欢个数 |
translation | string | 词霸小编 |
picture | string | 图片地址 |
picture2 | string | 大图片地址 |
caption | string | 标题 |
dateline | string | 时间 |
s_pv | string | 浏览数 |
sp_pv | string | 语音评测浏览数 |
tags | string | 相关标签 |
fenxiang_img | string | 合成图片 |
正常返回示例
1 |
|
登陆微信公众平台借口测试账号
微信公众号测试申请
https://mp.weixin.qq.com/debug/cgi-bin/sandbox?t=sandbox/login
扫描后手机端确认登陆
找到“新增测试模板”,添加模板消息
填写模板标题“每日一句”(可根据需求自己随便填)
提交后,记住改模版的id,一会用到。
找到测试二维码,扫描后,右侧出现你的昵称和微信号,记录下微信号
python程序
1 |
|
- 本文标题:Python 推送信息到wechat
- 本文作者:Roy
- 创建时间:2018-08-03 04:56:31
- 本文链接:https://www.yrzdm.com/2018/08/03/python2wx/
- 版权声明:本博客所有文章除特别声明外,均采用 BY-NC-SA 许可协议。转载请注明出处!